1. 빈(bean)이란?
JavaBeans : 재사용 가능한 컴포넌트, 상태(iv), getter&setter, no-args constructor
Servlet & JSP bean : MVC의 Model역활, EL, scope, JSP constainer가 관리
EJB Enterprise java Beans : 대기업에서 주로사용, 복잡한규칙, EJB container가 관리(관리란? 빈생성,소멸)
Spring Bean : POJO plain Old java Obect. 단순, 독립적, Spring container가 관리
2. BeanFactory와 ApplicationContext
Bean : Spring Container가 관리하는 객체
Spring container : Bean저장소, Bean을 저장, 관리 (생성, 소멸, 연결)
① BeanFactory : Bean을 생성, 연결 등의 기본기능을 정의해놓은 인터페이스 (빈을만드는 공장)
② ApplicationContext : BeanFactory를 확장해서 여러기능을 추가 정의해놓은 인터페이스
3. ApplicationContext의 종류
다양한 종류의 ApplicationContext 구현체를 제공※ 근래추세는 자바코드가 더많이 쓰인다. xml과 java코드 비슷하다.
예시) <bean> vs @bean
4. Root AC와 Servlet AC
5. Application Context의 주요메서드
빈 얻기 | |
object | getBean(Class<T> requiredType, Object...args) |
object | getBean(Class<T> requiredType) |
object | getBean(String name, Class<T> requiredType) |
object | getBean(String name, Object... args) |
object | getBean(String name) |
Singletone 인지 Prototype 인지 확인시 | |
boolean | isPrototype(String name) |
boolean | isSingletontype(String name) |
boolean |
isTypeMatch(String name, Class<T> typeToMatch)
|
boolean |
isTypeMatch(String name, ResolvableType typeToMatch)
|
bean이 있는지? | |
boolean | containsBean(String name) |
boolean | containsBeaDefinition(String neanName) |
boolean | containsLocalBean(String name) |
annotation이 있는지? | |
boolean |
findAnnotationOnBean(String beanName, classAnnotationType)
|
정의된 bean의 개수 | |
int | getBeanDefinitionCount() |
정의된 bean의 이름 | |
String[] | getBeanDefinitionNames() |
Root WebApplicationContext 가져오는 방법
package com.fastcampus.ch3;
import java.util.*;
import org.springframework.beans.factory.annotation.*;
import org.springframework.context.*;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.context.*;
import org.springframework.web.context.support.*;
import javax.servlet.*;
import javax.servlet.http.*;
@Controller
public class HomeController {
@Autowired
WebApplicationContext servletAC; // Servlet AC
@RequestMapping(value = "/", method = RequestMethod.GET)
public String home(Locale locale, HttpServletRequest request, Model model) {
// 원래는 request.getServletContext()지만, 컨트롤러는 HttpServlet을 상속받지 않아서 아래와 같이 해야함.
ServletContext sc = request.getSession().getServletContext(); // ApplicationContextFacade
WebApplicationContext rootAC = WebApplicationContextUtils.getWebApplicationContext(sc); // Root AC
System.out.println("webApplicationContext = " + rootAC);
System.out.println("servletAC = " + servletAC);
System.out.println("rootAC.getBeanDefinitionNames() = " + Arrays.toString(rootAC.getBeanDefinitionNames()));
System.out.println("servletAC.getBeanDefinitionNames() = " + Arrays.toString(servletAC.getBeanDefinitionNames()));
System.out.println("rootAC.getBeanDefinitionCount() = " + rootAC.getBeanDefinitionCount());
System.out.println("servletAC.getBeanDefinitionCount() = " + servletAC.getBeanDefinitionCount());
System.out.println("servletAC.getParent()==rootAC = " + (servletAC.getParent() == rootAC)); // servletAC.getParent()==rootAC = true
return "home";
}
}
로그분석
6-1. IoC와 DI
IoC (제어의 역전) : 제어의 흐름을 전통적인 방식과 다르게 뒤바꾸는것
DI (의존성 주입) : 사용할 객체를 외부에서 주입받는 것 ( 객체를 제공해주는 것 )
6-2. 스프링 애너테이션 - @Autowired (by Type)
@Autowired 적용 :
① 인스턴스 변수(iv),
② setter,
③ 참조형 매개변수를 가진 생성자,
④ 메서드
※ 생성자에는 @Autowired 생략가능
( 단, 생성자가 여러개일때는 @Autowired붙여야함. 왜? 여러개면 어느걸 사용할지 모르기때문에 )
Spring container에서 타입으로 bean을 검색해서 참조변수에 자동주입(DI)
검색된 bean이 n개이면, 그중에서 참조변수와 이름이 일치하는 것을 주입
주입 대상이 변수일 때, 검색된 bean이 1개 여야만 가능 ( 1개가 아니면 예외발생, required=false라면 0개도 가능)
주입 대상이 배열일 때, 검색된 bean이 n개 가능 ( 단, 0개면 예외발생 ! )
6-3. 스프링 애너테이션 - @Resource (by Name)
Spring container에서 이름으로 빈을 검색해서 참조변수에 자동주입(DI)
일치하는 이름의 bean이 없으면, 예외발생
6-3. 스프링 애너테이션 - @Component
<component-scan>으로 @Component가 클래스를 자동검색해서 빈으로 등록.
( <component-scan> : 패키지 지정시 아래 서브패키지 까지 모두 검색함 )
메타어노테이션이란 ? 어노테이션을 만들때 사용하는 어토네이션을 말함.
( 기본적으로 @Controller 어노테이션 내에, @Component 메타어노테이션이 이미 들어있음.
그래서 일전에 component-scan시 같이 자동검색됨
메타어노테이션이 들어가있는 것들 ( @Controller, @Service, @Repository, @ControllerAdvice의 메타어노테이션)
6-4. 스프링 애너테이션 - @Value, @PropertySource
@Value : 값을 지정할 때 사용
파일로부터 값을 읽어올수도 있다.
7. 스프링 어노테이션 vs 표준어노테이션 (JSR-330) JavaSpectRequest
8. bean의 초기화 : <property>와 setter

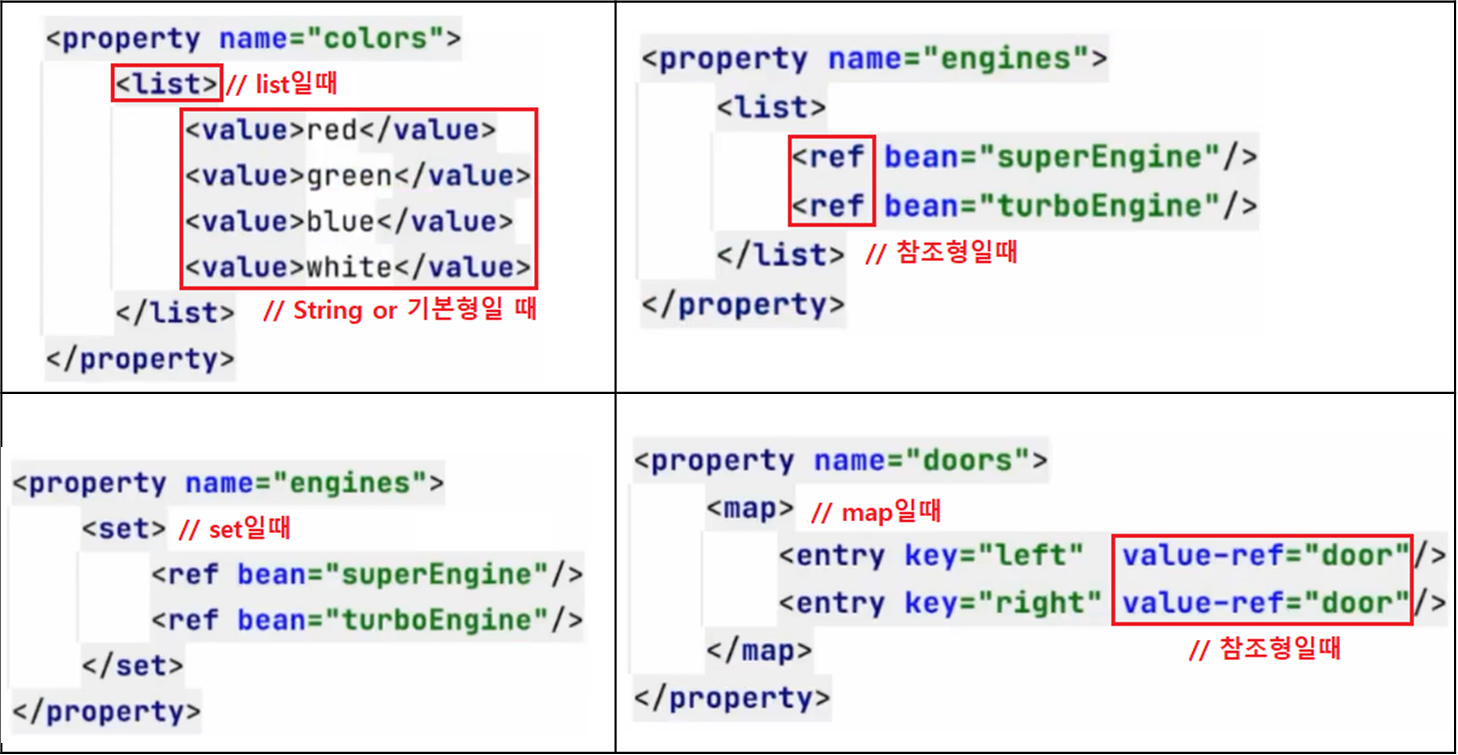
참고 :
남궁성, 스프링의 정석
'Spring의 정석' 카테고리의 다른 글
Spring_DI와 AOP(Spring 으로 DB연결)_13 (0) | 2023.03.23 |
---|---|
Spring_DI와 AOP(워크벤치 사용법, SQL 기초)_11 (0) | 2023.03.22 |
Spring_DI와 AOP(DI 활용하기 실습)_4 (0) | 2023.03.19 |
Spring_DI와 AOP(DI 맛보기)_1~3 (0) | 2023.03.17 |
SpringMVC 10 (DispatcherServlet, 데이터의 변환과검증) (0) | 2023.03.15 |